Laboratory Exercises
Below, there are the laboratory instructions from C programming. You can also practice ONLINE EXERCISES
Exercise 1:
The main purpose of this exercise is an introduction to C programming - writing simple programs, introduction to structure of the language, data types, definitions of constants, declarations of variables, arithmetic operators as well as instructions of assignments and data input and output.
1. Write a program that displays any text on the screen. The user should enter the displayed text from the keyboard.
2. Write a program that calculates a pole of any geometric figure.
3. Write a program that calculates an arithmetic average of any two numbers.
4. Write a program that verifies an identity of the following equation: (a+b)(a-b) = a²-b².
5. There are given the two legs of the right-angled triangle a and b. Calculate in degrees the hypotenuse and angles of the triangle.
6. Write a program that calculates a value of the following function:

Exercise 2:
The purpose of this exercise is to write programs that include bifurcations - conditional instructions, logical and relational operators and math functions from the standard library.
1. Write a program that calculates the sum of arbitrary n real numbers.
2. Write a program that returns the sum of the series with an accuracy of e=0.0001.
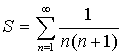
3. Calculate the variance σ² and and standard deviation

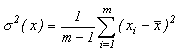

Practice tracing program execution!
4. Check whether all the natural numbers from the selected range 〈a, b〉 satisfy the inequality:

Print the number for which the inequality is not true.
5. Find the smallest natural number given with the following definition:
a.
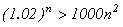
b.
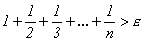
6. There is given a real number series terminated with zero. Zero is not included into the series. Calculate an arithmetic average of the positive numbers of the given series and return the quantity of numbers which absolute value is lower than 100.
Exercise 3:
Operations on the array type variables and formatting the results.
1. Write a program that puts into an array the names and surnames of n students as well as their addresses and prints the contents of this array on the screen in three columns. (n〈10).
2. Write a program that puts the elements of an A matrix of dimension n to k (n,k〈20) into an array and:
- Calculates and displays matrix of the sum of the row elements
- Calculates and displays matrix of the sum of the column elements
Exercise 4:
The main purpose of this exercise is an introduction to the structural programming - creating functions as well as practicing all programming skills gained so far.
1. There is given a function:
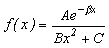
As long as the parameters A,B,C are positive, this function is decreasing. Write a program that tabulates the function above with the step specified by Delta x. Tabulating should be finished when the f(x) value decreases below the specified threshold ε.
The results should be printed on pages in two columns. Each single page should be printed after pressing any key.
2. Write a program that calculates the value of the expression W(n), while n represents the natural numbers.
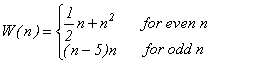
Change the program into a function, use it to write another program that writes into an array the following natural numbers within the range of 〈a, b〉 and their corresponding values of W(n). Print the results on the screen, using the pre-written procedure to display the results.
3. Write a program that verifies if particular number is the prime number. Change the program into a function and use it to write another program that puts the prime numbers into an array along with the numbers divisible by 23 and prints them on screen within the given range of 〈a, b〉.
Exercise 5:
The purpose of this exercise is to practice previously learnt elements of programming and control instructions such as switch, break, continue.
1. A twin prime is a prime number that differs from another prime number by two. Some examples of twin prime pairs are (3, 5), (5, 7), (11, 13), (17, 19), (29, 31) and (41, 43). Write a program that stores 20 twin primes larger than 'a' in an array and displays them on the screen. 'a' is any positive integer, entered with the the keyboard, smaller than 1000. The program should be immune to improper syntax (for example other characters entered) or to numbers with the values out of range.
2. Goldbach's conjecture says that every even number can be represented as the sum of two primes. Write a program that verifies this hypothesis for the first 20 even numbers.
3. Write a program that in user specified range 〈x1, x2〉 performs one with the three operations:
- designates prime numbers
- designates twin primes
- verifies Goldbach's conjecture
depending on the choice made by the user. The program should use a conditional statement switch.
Draw the block structure with the the internal blocks.
Exercise 6:
The purpose of this exercise is to get familiar with recursive functions, comparing different types of integers and real numbers and qualifiers of types.
1. Write a program that designates the factorial for any natural number n entered from the keyboard by the method chosen by the user. The program should have two functions to determine the factorial.
a. iterative method with the definitional formula: n! = 1*2*...*n
b. recursive method according to the definition: n! = n*(n-1)! where 0! = 1
Determine experimentally the maximum values of n for which it is possible to determine the factorial using all known types of integers and real numbers.
2. Write a program that for any n determines the function of 2n by a method chosen by the user. The program should have two functions to its determination:
- iterative method with the definitional formula: 2n = 2 * 2 *...* 2
- recursive method according to the definition: 2n = 2 * 2n-1 with 20 = 1
Determine the maximum value of n for the variable type used in the program.
3. Fibonacci Numbers are defined as follows:
x1 = 2, x2 = 5
xn = 2 xn-2 + xn-1, n = 3,4,5,...
a. Write a function which determines the first n Fibonacci numbers.
b. Use the function to write a program that calculates Fibonacci numbers for any range 〈k,l〉 entered from the keyboard.
4. Towers of Hanoi
It is a mathematical game or puzzle. It consists of three rods, and a number of disks of different sizes which can slide onto any rod. The puzzle starts with the disks in a neat stack in ascending order of size on one rod, the smallest at the top, thus making a conical shape.
The objective of the puzzle is to move the entire stack to another rod, obeying the following rules:
Only one disk may be moved at a time.
Each move consists of taking the upper disk from one of the rods and sliding it onto another rod, on top of the other disks that may already be present on that rod.
No disk may be placed on top of a smaller disk.
This problem is quite complex, but it is easy to formulate a recursive solution. In order to move n discs from stack A to B, initially one has to move n-1 discs from stack A to the secondary stack, move the n-th largest disk from A to B, then move n-1 discs from the secondary stack to stack B.
Write a program that for a given number of disks n that solves the problem of the towers of Hanoi.
Exercise 6:
The purpose of this exercise is to learn selected methods of array sorting and organizing large programs broken down into separate files, creating simple libraries, as well as practicing previously learnt elements of programming.
1. Write a program that generates 100 random integers in range 〈 1, 1000 〉 and stores them in an array of size 1x100, then sorts the numbers in a non-descending order using bubble sort (by simple substitution) and displays the result on the screen. The program should contain a separated bubble sort function.
2. Write a program that gets a sequence of n integers from the keyboard, inserts them into an array and displays them on the screen, then sorts the numbers in a non-descending order using insertion sort (by simple insertion) and displays the result on the screen. The program should contain a separate insertion sorting function.
3. Using the programs from the tasks 1 and 2, write a program that allows the user to enter the n numbers from the keyboard or generate them randomly. What is more, it allows the selection of the method of sorting and displays the input sequences and a sorted sequence in a format that the numbers are displayed in columns and fit the screen.
4. Write a the function for simple sorting and join it with the program from the third task.
5. Write a quicksort function and join it with the program written in tasks 1-4. Within the program, extract the file that contains all sort functions.
6. Write a program that puts into array the names and surnames of n students and sorts them alphabetically by their surnames (Polish letters are not to be taken into account). Choose any sort function.